Assignment 2
Python课业代写 For your second assignment, you will be asked to write python code to answer a series of questions. Your submission will comprise
For your second assignment, you will be asked to write python code to answer a series of questions. Your submission will comprise a single .txt file with all of your answers. Please make your answer to each question a separate function. To do so, name your response as follows:
def answer_to_question_<question number>(<arguments>):
You may use reuse functions by writing them separately as global functions. You should assume that I will be adding an if __name__ == ‘__main__’ section to the end of your code to test if it outputs the correct answer to a series of test cases. This means assume that I will be passing strings, lists, dictionaries, integers, etc. to your functions. Questions will specify the order in which data will be provided and the kinds of data they will contain. You are only required to provide code not code outputs. However, it is strongly recommended that you test your code with a few test cases prior to submission to ensure it works as intended.
Please note there will be grades both for your code comments and the readability of your code. Python课业代写
This means if you use functions are named it appropriately, etc. You will not be graded for how optimized your code runs. While good to do in practice, the main goal here is to see your ability to apply the concepts from class. Comments include a brief description of how your code works. You may do this either using # commented lines or the
“””
<your text here>
“””
multi-line string comment above. Comments are also important for receiving part marks for questions where your code does not provide the correct outputs.
You may import libraries, but you are responsible to ensure it will work with the expected data inputs and return the correct outputs. You may also use python editors. While I am not suggesting this be your choice, as an example Spyder is an editor that comes with anacondas.
You are welcome to use the jupyterhub server for the class or install python on your own machine. Responses are expected to be in python 3. Only code is required, not example outputs.
1.Area of a circle 1 pts Python课业代写
Write a program that receives as input the circumference of two circles as a list [float, float]. The program should output a list of the area of each circle and which circle is larger, with ties going to the first circle. EG) input: [2*3.14, 2*3.14] -> [3.14, 3.14, 0]
2.Hot Dog Calculator 1 pts
Assuming hot dogs come in packages of 10 and buns come in packages of 8, write a program to calculate the number of hot dog and bun packages needed for an integer input number of guests. Your output should specify [# hot dogs wasted, # buns wasted, # hot dog packages, # bun packages]
3.Restaurant selector 1.5 pts Python课业代写
Write a program to return a sorted list of acceptable restaurants based upon dietary restrictions. Your inputs will be dictionary{restaurant:[list of serviced diets]}, dictionary{patron: [list only of applicable diet restrictions]}. If no restaurant caters to the full list, return [‘No possible restaurant’] – note this is case sensitive.
4.Bug collector 1 pts
Write a program that receives a dictionary {integer day #: dictionary {bug type: integer}}. Output a list sorted by bug type the total number of bugs collected over all the days.
5.Temperature converter 1 pts Python课业代写
Write a loop that outputs a list of temperatures in Celsius for an input list of Fahrenheit temperatures. The formula to convert is F = 1.8 * C + 32.
6.Calculate the Fibonacci sum 1 pts
The Fibonacci sequence is defined as for the nth term F(n) = F(n-1) + F(n-2). The first few terms are [1,1,2,3,5,…}. Write a program that calculates the sum up to an input integer. EG) for n = 3 -> 1 + 1 + 2 = 4.
7.Calculating Pi 3 pts Python课业代写
Among many achievements, Sir Isaac Newton revolutionized the calculation of the number Pi. He did this by using the binomial theorem and calculus. This allowed him to calculate Pi more precisely than anyone in history in just a day (some people spent 20+ years on the calculation). You will now do the same calculation in a fraction of that time!
What you need:
The binomial theorem reads:
a) The binomial theorem – https://en.wikipedia.org/wiki/Binomial_theoremLinksLinks (https://en.wikipedia.org/wiki/Binomial_theoremLinksLinks) to an external site. to an external site.. You will use y = 1, x = x^2
b) n choose k formula – https://en.wikipedia.org/wiki/Binomial_coefficientLinksLinks (https://en.wikipedia.org/wiki/Binomial_coefficientLinksLinks) to an external site. to an external site., the formula is the first equation on the page.
c) The factorial formula – j! = j * (j-1) * (j-2) * … 2 * 1. EG) 2! = 2 * 1 = 2
d) Set n = 1/2 in the binomial theorem
e) The general equation for a circle of radius 1 is y^2 + x^2 = 1 => y = (1 – x^2)^0.5
f) For a circle of radius r = 1, the area of 1/4 of a circle = Pi /4
g) The area for 1/4 of a circle is the integral of (1 – x^2) ^ (0.5) dx between 0 and 1 (IE the integral of the top right quadrant of the circle)
h) The integral of x ^ n dx = x^(n+1) / n
i) Therefore, Pi/4 = The integral of (1 – x^2) ^ (0.5) dx from 0 to 1, where you can use the binomial expansion to make right hand integral easy to do for however many terms you want.
Using these formulas, write a program that for an input number of binomial expansion terms calculates the approximate value of Pi.
If you are interested, a summary of the problem and how Newton solved it can be found on youtube here: https://www.youtube.com/watch? v=gMlf1ELvRzc&ab_channel=VeritasiumLinksLinks (https://www.youtube.com/watch? v=gMlf1ELvRzc&ab_channel=VeritasiumLinksLinks) to an external site. to an external site. You are also welcome to use the further simplifications they discuss in the video (Newton ultimately integrated from 0 to 0.5 instead of 0 to 1 as it makes the calculation converge to Pi faster).
8.Creating an ADS in Pandas 1 pts Python课业代写
Using the tables from assignment 1, create an ADS, this time using Pandas. Then, take your code from assignment 1 and rewrite so that it would execute in the database from python – output not necessary for this question, only the code.
9.Calculating Inventory with Demand Floor 1.5 pts
Inventory at end of day = inventory from start of day + new supply – demand. Inventory at start of day = inventory at end of previous day. For two input dataframes calculate inventory at end of day by day from Jan 1 2021 to Dec 31 2021. Starting inventory on Jan 1 2021 = 0. Dataframes will be provided as [df[day, product, incoming supply], df[day, product, demand]].
1) The case where if inventory at end of previous day < 0 then inventory at start of day = 0. In other words, inventory cannot go below 0.
2) The case where inventory at end of previous day can go negative – IE excess demand carries forward to be filled at a later date.
Commenting your code 1.5 pts
Functions have comments that indicate what the function does, inputs with datatypes, outputs with datatypes, assumptions are explained, etc.
Code is legible 1.5 pts
Your code is well structured, easy to follow and it is clear how your code operates. Use the advice from lecture for a template on how to start this.
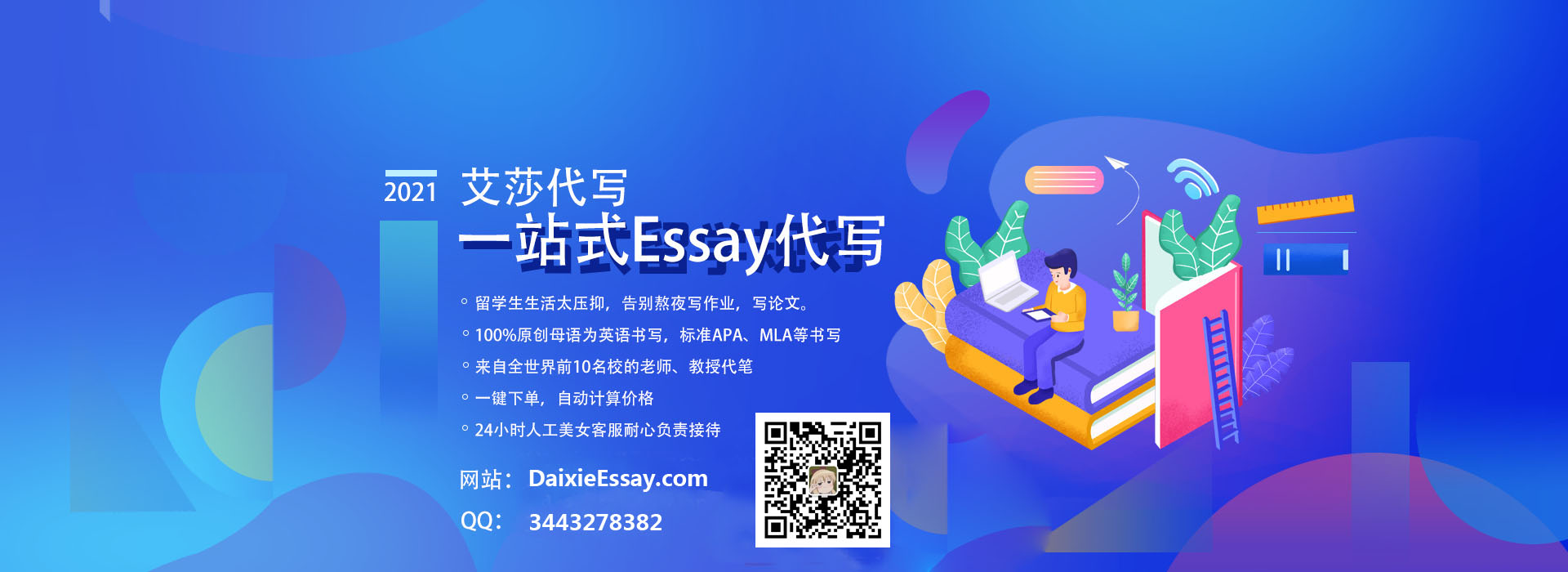
更多代写:数据库美国代上网课价格 雅思枪手 英国商科包网课 美国会计学assignment代写 美国文学网课代修 代写essay写作技巧